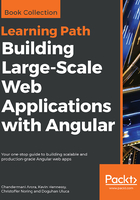
Workouts list views
To get the view working, open workouts.component.html and add the following markup:
<div class="row">
<div>
<abe-left-nav-main></abe-left-nav-main>
</div>
<div class="col-sm-10 builder-content">
<h1 class="text-center">Workouts</h1>
<div *ngFor="let workout of workoutList|orderBy:'title'" class="workout tile" (click)="onSelect(workout)">
<div class="title">{{workout.title}}</div>
<div class="stats">
<span class="duration" title="Duration"><span class="ion-md-time"></span> - {{(workout.totalWorkoutDuration? workout.totalWorkoutDuration(): 0)|secondsToTime}}</span>
<span class="float-right" title="Exercise Count"><span class="ion-md-list"></span> - {{workout.exercises.length}}</span>
</div>
</div>
</div>
</div>
We are using one of the Angular core directives, ngFor, to loop through the list of workouts and display them in a list on the page. We add the * sign in front of ngFor to identify it as an Angular directive. Using a let statement, we assign workout as a local variable that we use to iterate through the workout list and identify the values to be displayed for each workout (for example, workout.title). We then use one of our custom pipes, orderBy, to display a list of workouts in alphabetical order by title. We are also using another custom pipe, secondsToTime, to format the time displayed for the total workout duration.
Finally, we bind the click event to the following onSelect method that we add to our component:
onSelect(workout: WorkoutPlan) { this.router.navigate( ['/builder/workout', workout.name] ); }
This sets up navigation to the workout details page. This navigation happens when we click on an item in the workout list. The selected workout name is passed as part of the route/URL to the workout detail page.
Go ahead and refresh the builder page (/builder/workouts); one workout is listed, the 7 Minute Workout. Click on the tile for that workout. You'll be taken to the Workout screen and the workout name, 7MinWorkout, will appear at the end of the URL:
