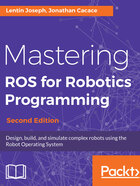
Interacting with V-REP using ROS topics
We will now discuss how to use topics to communicate with V-REP. This is useful when we want to send information to the objects of the simulation, or retrieve data generated by robots. While services are enabled when V-REP starts, topic communication happens only on demand, initializing publisher and subscriber variables in the simulation scene.
The most common way to program the simulation scene of V-REP is via Lua scripts. Every object of the scene can be associated to a script that is automatically invoked when a simulation starts and is cyclically executed during the simulation time.
In the next example, we will create a scene with two objects. One will be programmed to receive a float data from a specific topic, while the other one republishes the same data on another topic.
Use the drop-down menu on the Scene hierarchy panel, select the entry: Add | Dummy. We can create two objects, a dummy_publisher and a dummy_subscriber, and associate a script for each of them. Use the right mouse button on the created objects, and select the entry Add | Associated child script | Non-threaded, as shown in the following figure:

Alternatively, we can directly load the simulation scene by opening the demo_publisher_subscriber.ttt file located in the vrep_demo_pkg/scene directory. Let's see the content of the script associated to the dummy_subscriber object:
if (sim_call_type==sim_childscriptcall_initialization) then local moduleName=0 local moduleVersion=0 local index=0 local pluginNotFound=true while moduleName do moduleName,moduleVersion=simGetModuleName(index) if (moduleName=='Ros') then pluginNotFound=false end index=index+1 end if (pluginNotFound) then simDisplayDialog('Error','ROS plugin was not found.&&nSimulation will not run properly', sim_dlgstyle_ok, false ,nil,{0.8,0,0,0,0,0},{0.5,0,0,1,1,1}) else --Enable subscriber to /vrep_demo/float_in topic simExtROS_enableSubscriber("/vrep_demo/float_in",1,simros_strmcmd_set_float_signal ,-1,-1,"in") end end if (sim_call_type==sim_childscriptcall_actuation) then end if (sim_call_type==sim_childscriptcall_sensing) then end if (sim_call_type==sim_childscriptcall_cleanup) then end
Each Lua script linked to V-REP objects contains the following four sections:
- sim_childscriptcall_initialization: This section is executed only the first time that the simulation starts.
- sim_childscriptcall_actuation: This section is cyclically called at the same frame rate of the simulation. Users can put here the code that controls the actuation of the robot.
- sim_childscriptcall_sensing: This part will be executed in each simulation step, during the sensing phase of a simulation step.
- sim_childscriptcall_cleanup: This section is called just before the simulation ends.
Let's see the explanation of the preceding code:
if (sim_call_type==sim_childscriptcall_initialization) then local moduleName=0 local moduleVersion=0 local index=0 local pluginNotFound=true while moduleName do moduleName,moduleVersion=simGetModuleName(index) if (moduleName=='Ros') then pluginNotFound=false end index=index+1 end
In the initialization part, we check if the vrep_plugin is installed in the system, otherwise, an error is displayed:
simExtROS_enableSubscriber("/vrep_demo/float_in",1,simros_strmcmd_set_float_signal ,-1,-1,"in")
This activates the subscriber of the input float value on the /vrep_demo/float_in topic. The simExtROS_enableSubscriber function expects as parameters the name of the topic, the desired queue size, the desired type to stream, and three enabling parameters. These parameters specify the item upon which the data should be applied. For example, if we want to set the position of a joint object, the first parameter will be the object handle, while the other parameters will not be used. In our case, we want to save the value received from the topic into the variable "in".
Let's now see the content of the script associated to the dummy_publisher object:
if (sim_call_type==sim_childscriptcall_initialization) then -- Check if the required plugin is there (libv_repExtRos.so or libv_repExtRos.dylib): local moduleName=0 local moduleVersion=0 local index=0 local pluginNotFound=true while moduleName do moduleName,moduleVersion=simGetModuleName(index) if (moduleName=='Ros') then pluginNotFound=false end index=index+1 end if (pluginNotFound) then simDisplayDialog('Error','ROS plugin was not found.&&nSimulation will not run properly', sim_dlgstyle_ok, false, nil, {0.8,0,0,0,0,0},{0.5,0,0,1,1,1}) else simExtROS_enablePublisher("/vrep_demo/float_out", 1,simros_strmcmd_get_float_signal, -1,-1,"out") end end end if (sim_call_type==sim_childscriptcall_actuation) then --Get value of input signal and publish it on /vrep_demo/float_out topic data = simGetFloatSignal('in') if( data ) then simSetFloatSignal("out",data) simAddStatusbarMessage(data) end end if (sim_call_type==sim_childscriptcall_sensing) then -- Put your main SENSING code here end if (sim_call_type==sim_childscriptcall_cleanup) then -- Put some restoration code here End
The code is explained here:
simExtROS_enablePublisher("/vrep_demo/float_out", 1,simros_strmcmd_get_float_signal, -1,-1,"out")
In this line, after checking the correct installation of the vrep_plugin, we will enable the publisher of the float value. After this line, the script publishes continuously the value of the variable "out":
if (sim_call_type==sim_childscriptcall_actuation) then --Get value of input signal and publish it on /vrep_demo/float_out topic data = simGetFloatSignal('in') if( data ) then simSetFloatSignal("out",data) simAddStatusbarMessage(data) end end
Finally, we set the value of the out variable with the one received from the /vrep_demo/float_in topic, stored in the in variable. Note that in and out are special global variables accessible from all scripts of the scene. These variables in V-REP are called signals.
After running the simulator, we can check that everything works correctly, publishing a desired number on the input topic and monitoring the output from the output topic, using the following commands:
$ rostopic pub /vrep_demo/float_in std_msgs/Float32 "data: 2.0" -r 12
$ rostopic echo /vrep_demo/float_out
