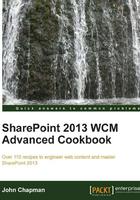
Importing a design package to all site collections with PowerShell
Applying a design package to a large number of site collections can be a tedious task. To expedite the process, we can use PowerShell. In this recipe, we are going to use a PowerShell script (PS1) to upload and apply a design package to each content site collection in each web application on the local SharePoint farm.
How to do it...
Follow these steps to import a design package to all site collections with PowerShell:
- Open your preferred text editor to create the PS1 script file.
- Load the
Microsoft.SharePoint.dll
andMicrosoft.SharePoint.Publishing.dll
assemblies into the PowerShell session.[Reflection.Assembly]::LoadFrom("C:\Program Files\Common Files\microsoft shared\Web Server Extensions\15\ISAPI\Microsoft.SharePoint.Publishing.dll")
[Reflection.Assembly]::LoadFrom("C:\Program Files\Common Files\microsoft shared\Web Server Extensions\15\ISAPI\Microsoft.SharePoint.dll")
- Specify the path to the design package WSP file and get the filename from the path.
$filePath = "C:\My PowerShell Design-1.0.wsp"
$fileName = [System.IO.Path]::GetFileName($filePath)
- Create a
DesignPackageInfo
object to represent the design package we are about to upload. In the constructor, specify the major and minor versions of the design package.$package = New-Object Microsoft.SharePoint.Publishing.DesignPackageInfo($fileName, [Guid]::Empty, 1, 0)
- Create a temporary folder name to upload the design package in each site collection.
$tempFolderName = "temp_designupload_" + ([Guid]::NewGuid).ToString()
- Use the
OpenRead
method ofSystem.IO.File
to read the contents of the design package WSP file and add the file to theFiles
collection of the temporary folder.$fileBinary = [System.IO.File]::OpenRead($filePath)
- Use a
foreach
loop to iterate through each contentSPWebApplication
on the local SharePoint farm using theGet-SPWebApplication
Cmdlet.foreach($webApp in (Get-SPWebApplication))
- Use a
foreach
loop to iterate through eachSPSite
Cmdlet in theSites
property of theSPWebApplication
object.foreach($site in $webApp.Sites)
- Verify the
CompatibilityLevel
property of theSPSite
object to ensure it is in SharePoint 2013 (Version 15) mode and not in SharePoint 2010 (Version 14) mode.if ($site.CompatibilityLevel –eq 15)
- Using the following command, create a temporary folder in the
RootWeb
site to upload the design package:$tempFolder = $site.RootWeb.RootFolder.SubFolders.Add($tempFolderName)
- Add the file to the
Files
collection of the temporary folder.$file = $tempFolder.Files.Add($fileName, $fileBinary, $true)
- Use the
Install
method ofMicrosoft.SharePoint.Publishing.DesignPackage
to add the design package to the Solutions Gallery and apply the customizations in the design package to the site collection.[Microsoft.SharePoint.Publishing.DesignPackage]::Install($site, $package, $file.Url)
- Delete the temporary folder.
$tempFolder.Delete()
- After the
foreach
loops are completed, close the design package WSP file.$fileBinary.Close()
- Use the
Dispose
method to discard theSPSite
object.$site.Dispose()
- Save the file as a PS1 file, for example,
importdesignpackage.ps1
. - Execute the script in the PowerShell session.
./importdesignpackage.ps1
How it works...
PowerShell provides a scripting environment that can simplify repetitive administrative tasks. Using PowerShell, we are able to use a combination of the Cmdlets provided and the .NET code to iterate through each site collection in each web application to import and apply our design package.
In this recipe, we used the Get-SPWebApplication
Cmdlet to retrieve all of the content web applications on the local SharePoint farm. We then iterated through each site collection in the Sites
property of each web application. For each site collection, we uploaded the design package to a temporary folder. Lastly, we installed the design package to each site collection from the temporary folder.
There's more...
This recipe may also be accomplished with code using the server-side object model.
Follow these steps to import and apply a design package to all site collections using the server-side object model:
- Specify the path to the design package WSP file and get the filename from the path.
var filePath = "C:\My Code Design-1.0.wsp"; var fileName = Path.GetFileName(filePath);
- Create a
DesignPackageInfo
object to represent the design package we are about to upload. In the constructor, specify the major and minor versions of the design package.var package = new DesignPackageInfo(fileName, Guid.Empty, 1, 0);
- Create a temporary folder name to upload the design package in each site collection.
var tempFolderName = "temp_designupload_" + Guid.NewGuid().ToString();
- Use the
OpenRead
method ofSystem.IO.File
to read the contents of the design package WSP file and add the file to theFiles
collection of the temporary folder.var fileBinary = File.OpenRead(filePath);
- Use a
foreach
loop to iterate through each contentSPWebApplication
on the local SharePoint farm.foreach(var webApp in SPWebService.ContentService.WebApplications)
- Use a
foreach
loop to iterate through eachSPSite
in theSites
property of theSPWebApplication
object.foreach(SPSite site in webApp.Sites)
- Verify the
CompatibilityLevel
property of theSPSite
object to ensure it is in SharePoint 2013 (Version 15) mode and not in SharePoint 2010 (Version 14) mode.if (site.CompatibilityLevel == 15)
- Create a temporary folder in the
RootWeb
site to upload the design package to.var tempFolder = site.RootWeb.RootFolder.SubFolders.Add(tempFolderName);
- Add the file to the
Files
collection of the temporary folder.var file = tempFolder.Files.Add(fileName, fileBinary, true);
- Use the
Install
method ofDesignPackage
to add the design package to the Solutions Gallery and apply the customizations in the design package to the site collection.DesignPackage.Install(site, package, file.Url);
- Delete the temporary folder.
tempFolder.Delete();
- Discard the
SPSite
object using theDispose
method.site.Dispose();
- After the
foreach
loops are completed, close the design package WSP file.fileBinary.Close();
See also
- The SharePoint 2013 Design Manager design packages article on MSDN at http://msdn.microsoft.com/en-us/library/jj862342.aspx
- The How to: Upload a File to a SharePoint Site from a Local Folder article on MSDN at http://msdn.microsoft.com/en-us/library/ms454491(v=office.14).aspx
- The SPWeb class topic on MSDN at http://msdn.microsoft.com/en-us/library/Microsoft.SharePoint.SPWeb.aspx
- The SPSite class topic on MSDN at http://msdn.microsoft.com/en-us/library/microsoft.sharepoint.spsite.aspx
- The Get-SPWebApplication topic on TechNet at http://technet.microsoft.com/en-us/library/ff607562.aspx